net.rim.device.api.smartcard
Class SmartCardReader
java.lang.Object
net.rim.device.api.smartcard.SmartCardReader
public abstract class SmartCardReader
- extends Object
Represents a physical smart card reader.
Note to implementors: Most, if not all, UI functionality has been implemented in the base class.
Typically, subclasses should not need to implement any UI.
To communicate with the reader, the developer must call openSession()
.
For information on implementing smart card readers, see Adding smart card reader drivers to the SmartCard API.
This tutorial describes the process of adding support for new smart card reader and smart card reader drivers to the API. A smart card reader consists of the
physical device and the software driver used to read the data from a smart card.
- See Also:
SmartCardReaderSession
,
SmartCardReaderFactory
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
Constructor Summary |
|
protected |
SmartCardReader()
Constructs a SmartCardReader. |
SmartCardReader
protected SmartCardReader()
- Constructs a SmartCardReader.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
openSession
public final SmartCardReaderSession openSession()
throws SmartCardException
- Returns a communication session with the reader.
The user may be prompted to attach the reader, if the reader could not be found.
For security reasons, only only one communication session is allowed to be open with a reader at one time.
Hence, this function will block until all other communication sessions using this reader have closed.
- Returns:
- A smart card reader communication session.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the smart card.
SmartCardCancelException
- Thrown if the user presses cancel when asked to install their reader.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
openSessionImpl
protected abstract SmartCardReaderSession openSessionImpl()
throws SmartCardException
- Returns the appropriate subclass of
SmartCardReaderSession
.
Implementations of the method should not bring up UI.
- Returns:
- The
SmartCardReaderSession
subclass.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the smart card.
SmartCardNoReaderPresentException
- Thrown if the reader is not installed.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isReaderPresent
public final boolean isReaderPresent()
throws SmartCardException
- Returns true if the reader is attached, and false otherwise.
The implementation of this function should not bring up UI.
The implementation of this function must not call
openSession()
.
- Returns:
- A boolean that determines if the reader is attached.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the
smart card.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isReaderPresentImpl
protected abstract boolean isReaderPresentImpl()
throws SmartCardException
- Returns true if the reader is attached, and false otherwise.
The implementation of this function should not bring up UI.
The implementation of this function must not call
openSession()
.
- Returns:
- A boolean that determines if the reader is attached.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the
smart card.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isSmartCardPresent
public final boolean isSmartCardPresent()
throws SmartCardException
- Returns true if a smart card is present in the reader, and false
otherwise.
Should return false when there is not reader present.
The implementation of this function should not bring up UI.
The implementation of this function must not call openSession().
- Returns:
- A boolean that determines if the smart card is present in the
reader.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the
smart card.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isSmartCardPresentImpl
protected abstract boolean isSmartCardPresentImpl()
throws SmartCardException
- Returns true if a smart card is present in the reader, and false
otherwise.
Should return false when there is not reader present.
The implementation of this function should not bring up UI.
The implementation of this function must not call openSession().
- Returns:
- A boolean that determines if the smart card is present in the
reader.
- Throws:
SmartCardException
- Thrown if an error occurs while reading the
smart card.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isInsertionRemovalDetectable
public final boolean isInsertionRemovalDetectable()
- Returns true if the reader can detect when a card is inserted in or removed
from the reader.
Some readers may recognize when a card is inserted or removed. If this functionality
is not supported by the reader, then this method must returns false.
- Returns:
- true if the reader can detect when a card is inserted or removed.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isInsertionRemovalDetectableImpl
protected abstract boolean isInsertionRemovalDetectableImpl()
- Returns true if the reader can detect when a card is inserted in or removed
from the reader.
Some readers may recognize when a card is inserted or removed. If this functionality
is not supported by the reader, then this method must returns false.
- Returns:
- true if the reader can detect when a card is inserted or removed.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
getLabel
public final String getLabel()
- Returns a label associated with the kind of smart card reader.
The String should not include the words "smart card reader", as this method will
be used to generate strings such as ( "Please insert your %s smart card
reader", getLabel() )
- Returns:
- A String representing the label of the smart card reader.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
getLabelImpl
protected abstract String getLabelImpl()
- Returns a label associated with the kind of smart card reader.
The String should not include the words "smart card reader", as this method will
be used to generate strings such as ( "Please insert your %s smart card
reader", getLabel() )
- Returns:
- A String representing the label of the smart card reader.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
getType
public final String getType()
- Returns the type of the reader, eg "Serial Port" or "Bluetooth".
A session does not need to be open to get a valid response from this method.
- Returns:
- A String representation of the type of the reader.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
getTypeImpl
protected abstract String getTypeImpl()
- Returns the type of the reader, eg "Serial Port", "Bluetooth".
A session does not need to be open to get a valid response from this method.
- Returns:
- A String representation of the type of the reader.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
addListener
public final boolean addListener(ReaderStatusListener listener)
- Registers a
ReaderStatusListener
with this reader.
Some readers may recognize when a card is inserted or removed. If this functionality
is not supported by the reader, then this method returns false.
If smart card insertions and removals are recognized, then when addListener is called,
listener.readerStatus() will be called directly with either ReaderStatus.CARD_PRESENT,
ReaderStatus.CARD_ABDSENT, or ReaderStatus.CARD_UNKNOWN if there was an exception.
When there is a change in the reader status, for example if the card is inserted or removed
listener.readerStatus() will be called with either ReaderStatus.CARD_INSERTED or
ReaderStatus.CARD_REMOVED.
- Parameters:
listener
- The listener to add.
- Returns:
- true if card insertions and removals are detectable and the listener was successfully added.
Returns false if card insertions and removals are not detectable.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
removeListener
public final boolean removeListener(ReaderStatusListener listener)
- Unregisters the given listener with this reader.
- Parameters:
listener
- The listener to be unregistered from the reader.
- Returns:
- true if the listener was removed, false otherwise.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
setReaderStatus
protected final void setReaderStatus(int readerStatus)
- Called by the subclass implementation to notify all registered
ReaderStatusListeners
with the given status.
Note: all Throwable exceptions thrown by the listeners are ignored.
- Parameters:
readerStatus
- An integer representing the status of the reader.- See Also:
ReaderStatus
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
getReaderStatus
public final int getReaderStatus()
- Returns the current reader status.
- Returns:
- An integer representing the reader status.
The state diagram represents the different possible paths to a return value.
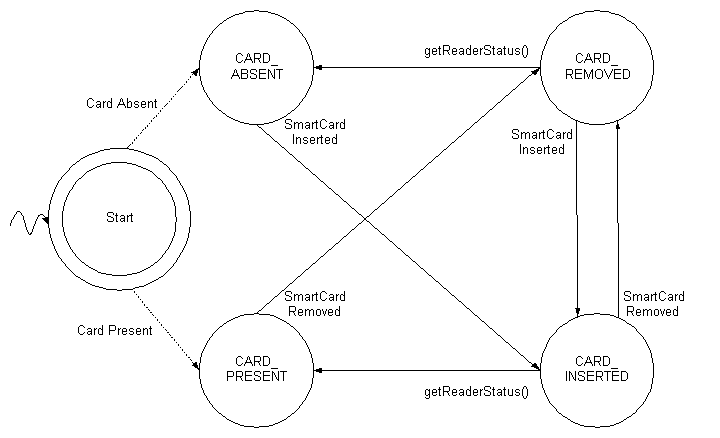
- See Also:
ReaderStatus
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
displaySettings
public final void displaySettings(Object context)
- Allows the driver to display some settings or properties.
This method will be invoked from the smart card options screen when
the user selects the driver and chooses to view the settings of that driver.
This method could be called from the event thread. The driver should not block
the event thread for long periods of time.
- Parameters:
context
- Reserved for future use.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
displaySettingsImpl
protected void displaySettingsImpl(Object context)
- Allows the driver to display some settings or properties.
This method will be invoked from the smart card options screen when
the user selects the driver and chooses to view the settings of that driver.
This method could be called from the event thread. The driver should not block
the event thread for long periods of time.
- Parameters:
context
- Reserved for future use.- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isDisplaySettingsAvailable
public final boolean isDisplaySettingsAvailable(Object context)
- Allows the driver to indicate if they support displaying settings.
If this method returns true, then the method displaySettings maybe called
to show settings to the user.
- Parameters:
context
- Reserved for future use.
- Returns:
- true if the driver supports displaying settings, false otherwise.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
isDisplaySettingsAvailableImpl
protected boolean isDisplaySettingsAvailableImpl(Object context)
- Allows the driver to indicate if they support displaying settings.
If this method returns true, then the method displaySettings maybe called
to show settings to the user.
- Parameters:
context
- Reserved for future use.
- Returns:
- true if the driver supports displaying settings, false otherwise.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
toString
public String toString()
- Description copied from class:
Object
- Returns a string representation of the object. In general, the
toString
method returns a string that
"textually represents" this object. The result should
be a concise but informative representation that is easy for a
person to read.
It is recommended that all subclasses override this method.
The toString
method for class Object
returns a string consisting of the name of the class of which the
object is an instance, the at-sign character `@
', and
the unsigned hexadecimal representation of the hash code of the
object. In other words, this method returns a string equal to the
value of:
getClass().getName() + '@' + Integer.toHexString(hashCode())
- Overrides:
toString
in class Object
- Returns:
- a string representation of the object.
- Category:
- Signed: This element is only accessible by signed applications. If you intend to use this element, please visit http://www.blackberry.com/go/codesigning to obtain a set of code signing keys. Code signing is only required for applications running on BlackBerry smartphones; development on BlackBerry Smartphone Simulators can occur without code signing.
- Since:
- BlackBerry API 4.1.0
Copyright 1999-2011 Research In Motion Limited. 295 Phillip Street, Waterloo, Ontario, Canada, N2L 3W8. All Rights Reserved.
Java is a trademark of Oracle America Inc. in the US and other countries.
Legal